반응형
도전 Mission!
1. while문을 이용하여 구구단 5단 출력
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>while문을 이용한 구구단 5단</title>
</head>
<body>
<script>
var i = 1;
document.write("<h2>구구단 5단</h2>")
while(i < 10) {
document.write("5 X " + i + " = " + i * 5 + "</br>");
i++
}
</script>
</body>
</html>
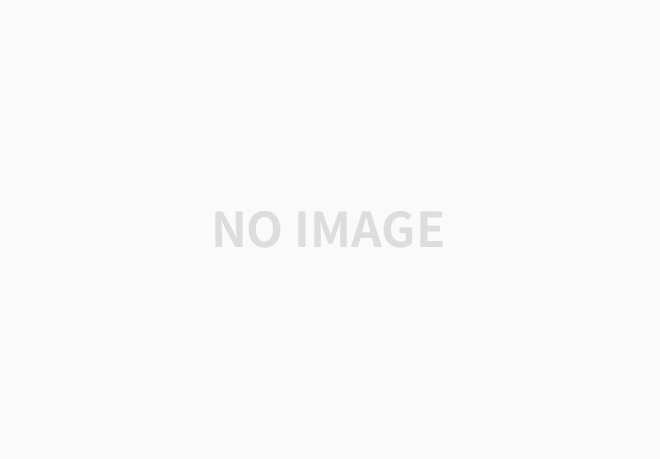
2. 중첩 for문을 이용하여 5행 5열 표 만들기
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>중첩 for문을 이용한 5행 5열 표 만들기</title>
</head>
<body>
<script>
var num = 1;
var t = "<table border=1>";
for(var i=1; i<=5; i++) {
t += "<tr>";
for(var j=1; j<=5; j++) {
t+="<td>" + num + "</td>"
num++;
}
t += "</tr>";
}
t += "</table>";
console.log(t);
document.write(t);
</script>
</body>
</html>
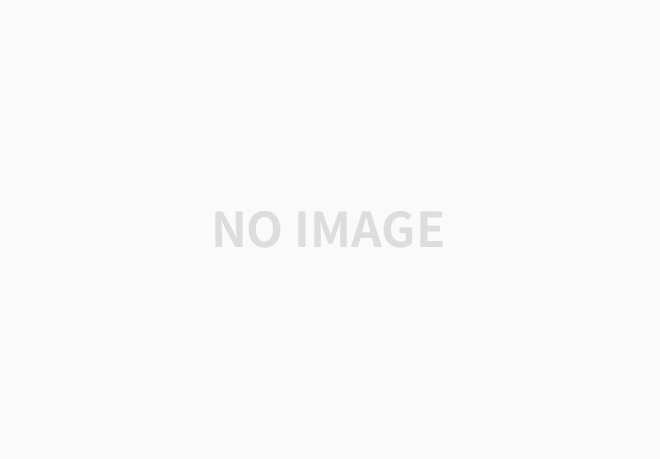
homework
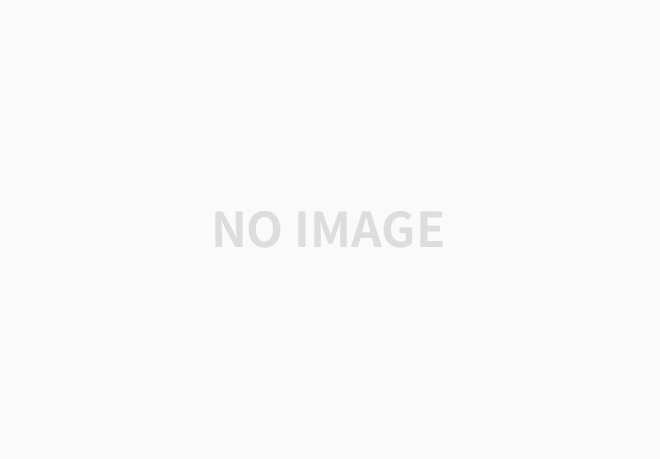
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>짝수일까, 홀수일까</title>
<style>
body {
text-align: center;
}
</style>
</head>
<body>
<h2>짝수일까, 홀수일까</h2>
<script>
var num = prompt("숫자를 입력하시오.");
var result;
if(num % 2 == 0) {
result = "짝수";
} else {
result = "홀수";
}
document.write(num + "는 " + result + "입니다.");
//삼항 연산자
// var result2 = num % 2 == 0 ? "는(은) 짝수입니다." : "는(은) 홀수입니다.";
// document.write(num + result2);
</script>
</body>
</html>
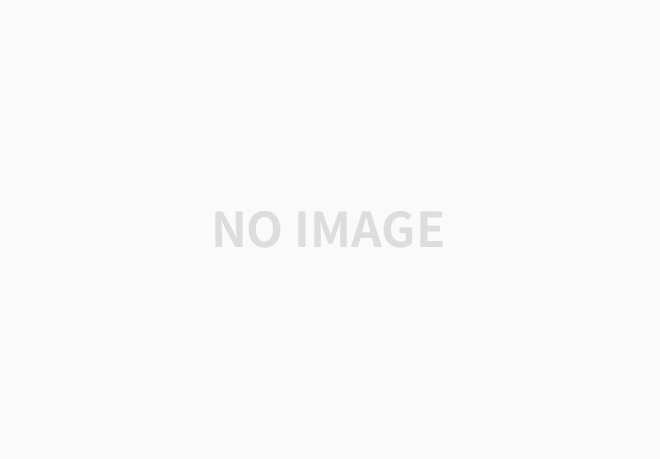
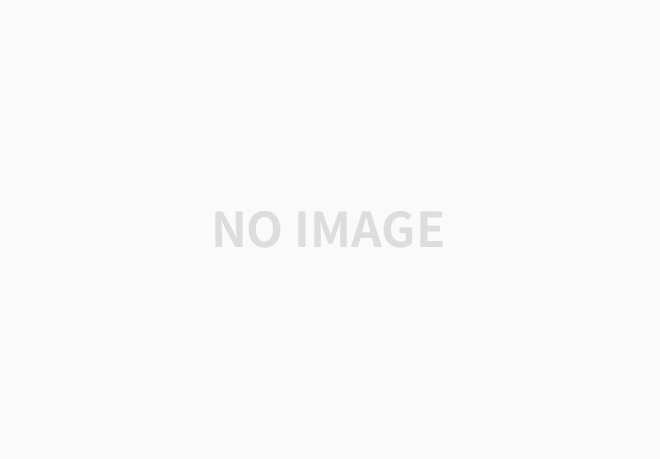
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>3의 배수 찾기</title>
<style>
body {
font-size:1.2em;
text-align:center;
}
</style>
</head>
<body>
<h2>3의 배수 찾기</h2>
<script>
// 1. for문과 continue문 사용
var count = 0;
for(var i=1; i<=100; i++) {
if(i % 3 != 0) {
continue;
} document.write(i + ", ");
i++;
count++;
} document.write("<br><br>3의 배수의 갯수 : " + count);
// 2. while문과 continue문 사용
// while문안에 continue문 쓸 때 반드시 첫 째줄에는 증감식이 와야 한다!!
var i = 0;
var count = 0;
while(i <= 100) {
i++;
if(i % 3 != 0) {
continue;
} document.write(i + ", ");
count++;
}
document.write("<br><br>3의 배수의 갯수 : " + count);
// 3. for문과 if문 사용
var count = 0;
for(var i = 1; i <= 100; i++) {
if(i % 3 == 0) {
document.write(i, ", ");
count++;
}
} document.write("<br><br>3의 배수의 갯수 : ", count);
</script>
</body>
</html>
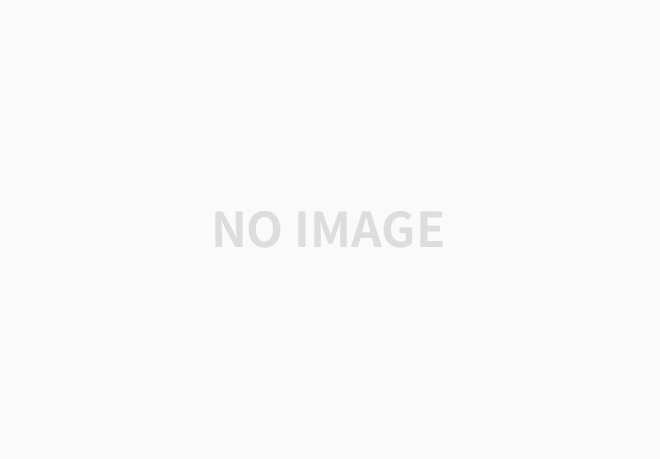
3. 별찍기 (증가)
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>별 찍기 (증가)</title>
</head>
<body>
<h6>별 찍기 (증가)</h6>
<script>
for(var i = 1; i <= 5; i++) {
for(var j = 1; j <= i; j++) {
document.write("*");
} document.write("<br>")
}
</script>
</body>
</html>
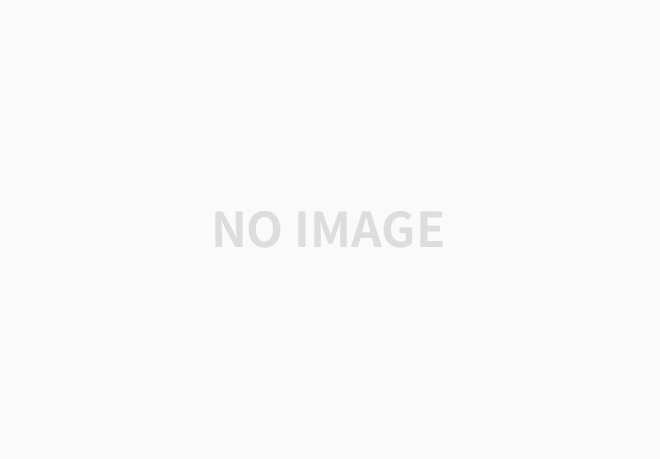
4. 별찍기 (감소)
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>별 찍기 (감소)</title>
</head>
<body>
<h6>별 찍기 (감소)</h6>
<script>
for(var i = 1; i <= 5; i++) {
for(var j = 1; j <= 6 - i; j++) {
document.write("*");
} document.write("<br>")
}
</script>
</body>
</html>
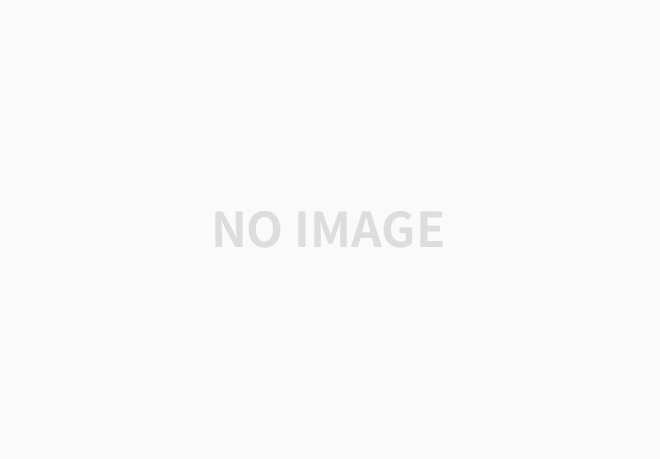
5. 별 찍기 (모래시계)
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>별 찍기 (모래시계)</title>
</head>
<body>
<h6>별 찍기 (모래시계)</h6>
<script>
for(var i = 1; i <= 5; i++) {
for(var j = 1; j <= i; j++) {
document.write(" ");
}
for(var j = 1; j <= 11 - 2 * i ; j++) {
document.write("*");
} document.write("<br>")
}
for(var i = 1; i <= 5; i++) {
for(var j = 1; j <= 5 - i; j++) {
document.write(" ");
}
for(var j = 1; j <= 2 * i + 1; j++) {
document.write("*");
} document.write("<br>")
}
</script>
</body>
</html>
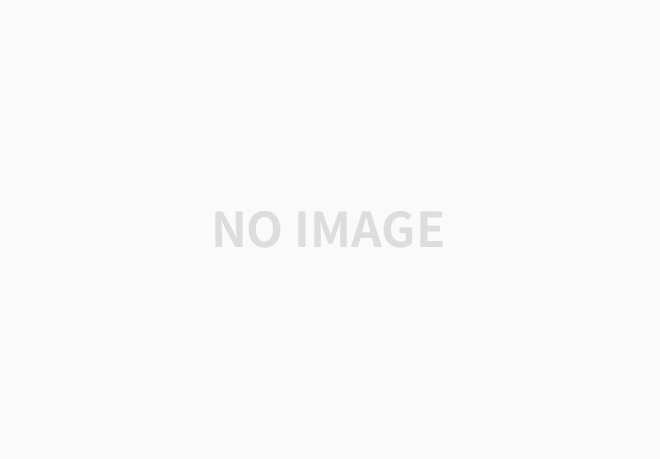
반응형
'🤼♂️ 5. Project > 5-2 연습문제 풀이' 카테고리의 다른 글
[Do it! 자바스크립트+제이쿼리 입문] 5장 함수 (0) | 2021.09.12 |
---|---|
[Do it! 자바스크립트+제이쿼리 입문] 4장 객체 (0) | 2021.09.12 |
[Do it! 자바스크립트+제이쿼리 입문] 2장 자바스크립트 기초 문법 (0) | 2021.09.12 |
[Do it! 자바스크립트+제이쿼리 입문] 1장 자바스크립트 시작하기 (0) | 2021.09.05 |
[혼자공부하는자바] 14장 입출력 스트림 (0) | 2021.09.05 |
댓글